목록Development/Algorithm & Coding Test (189)
컴공생의 다이어리
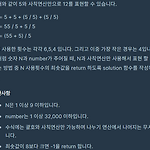
[프로그래머스] N으로 표현 - 파이썬(Python) def solution(N, number): if N == number: return 1 dp = [{N}] for i in range(2, 9): # 숫자 n을 i번 사용할 때 nums = set([int(str(N) * i)]) # 기본으로 숫자 n을 i번 이어서 생성 for j in range(0, int(i / 2)): for x in dp[j]: # n을 j+1번 사용했을 때의 경우와 for y in dp[i - j - 2]: # n을 i-j-1번 사용했을 때의 경우 nums.add(x + y) nums.add(x - y) nums.add(y - x) nums.add(x * y) if y != 0: nums.add(x // y) if x !=..
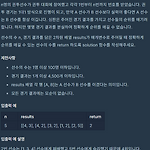
[프로그래머스] 순위 - 파이썬(Python) from collections import defaultdict def solution(n, results): answer = 0 win, lose = defaultdict(set), defaultdict(set) for w, l in results: win[l].add(w) # 나를 이긴 사람 lose[w].add(l) # 나에게 진 사람 for i in range(1, n + 1): for w in win[i]: lose[w].update(lose[i]) for l in lose[i]: win[l].update(win[i]) for i in range(1, n + 1): if len(win[i]) + len(lose[i]) == n - 1: answer ..
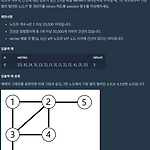
[프로그래머스] 가장 먼 노드 - 파이썬(Python) from collections import deque def bfs(graph, start, n): visited = [0] * (n + 1) visited[start] = 1 queue = deque([start]) while queue: node = queue.popleft() for i in graph[node]: if visited[i] == 0: visited[i] = visited[node] + 1 queue.append(i) return visited.count(max(visited)) def solution(n, vertex): graph = [[] for _ in range((n + 1))] for a, b in vertex: gra..
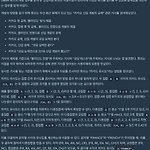
[프로그래머스] 뉴스 클러스터링 - 파이썬(Python) from collections import Counter def solution(str1, str2): # 소문자로 변경 str1, str2 = str1.lower(), str2.lower() # 문자열 조각들 만들기 str1_set = list(str1[i:i + 2] for i in range(len(str1) - 1) if str1[i:i + 2].isalpha()) str2_set = list(str2[i:i + 2] for i in range(len(str2) - 1) if str2[i:i + 2].isalpha()) # 문자열 조각들 세기 str1_counter, str2_counter = Counter(str1_set), Counter..
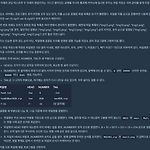
[프로그래머스] 파일명 정렬 - 파이썬(Python) def solution(files): answer = [] data = [] # 정렬할 파일명 정보 저장 len_files = len(files) # 파일 수 for i in range(len_files): HEAD, NUMBER = '', '' for j in files[i]: if not j.isdigit() and not NUMBER: # HEAD 부분 HEAD += j.lower() elif j.isdigit() and HEAD: # NUMBER 부분 NUMBER += j else: # TAIL 부분 break data.append((HEAD, NUMBER, i)) data.sort(key=lambda x: (x[0], x[1], x[2])) ..
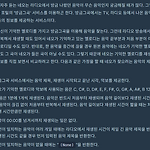
[프로그래머스] 방금그곡 - 파이썬(Python) def shap_to_lower(s): # 샵이 포함된 음을 소문자로 변경 s = s.replace('C#', 'c').replace('D#', 'd').replace('F#', 'f').replace('G#', 'g').replace('A#', 'a') return s def solution(m, musicinfos): data = [] m = shap_to_lower(m) for i in musicinfos: i = i.split(',') data.append([ int(i[1][:2]) * 60 + int(i[1][3:]) - (int(i[0][:2]) * 60 + int(i[0][3:])), # 재생시간 i[2], # 제목 shap_to_lower..
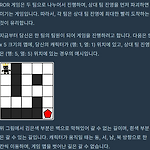
[프로그래머스] 게임 맵 최단거리 - 파이썬(Python) from collections import deque def solution(maps): len_x, len_y = len(maps), len(maps[0]) queue = deque([(0, 0)]) directions = [(1, 0), (-1, 0), (0, 1), (0, -1)] # 상하좌우 while queue: # bfs 수행 x, y = queue.popleft() for i in range(4): xx = x + directions[i][0] yy = y + directions[i][1] if 0
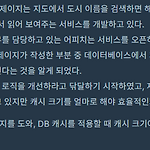
[프로그래머스] 캐시 - 파이썬(Python) from collections import deque def solution(cacheSize, cities): answer = 0 cache = deque(maxlen=cacheSize) if cacheSize == 0: # 캐시 크기가 0일 때 return len(cities) * 5 for c in cities: c = c.lower() # 대소문자 구분하지 않기 위해 모두 소문자로 변경 if c in cache: # 캐시에 있는 데이터라면 answer += 1 cache.remove(c) # 데이터 삭제 else: # 캐시에 없는 데이터라면 answer += 5 cache.append(c) return answer https://programmers...
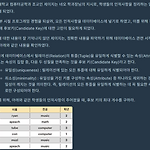
[프로그래머스] 후보키 - 파이썬(Python) from itertools import combinations def solution(relation): len_key = len(relation[0]) candidate_key = [] for i in range(1, len_key + 1): for combi in combinations(range(len_key), i): # 후보키가 될수 있는 key들의 전체 조합 temp = list() for r in relation: curr = [r[c] for c in combi] # 현재 후보키 조합에 해당하는 튜플 데이터 if curr in temp: # 유일성을 만족하지 않는 경우 break else: temp.append(curr) else: for c..
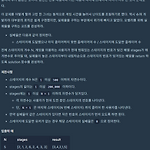
[프로그래머스] 실패율 - 파이썬(Python) import collections def solution(N, stages): total_people = len(stages) stage_count = dict(collections.Counter(stages)) result = [] for i in range(1, N + 1): if i in stage_count.keys(): result.append((i, stage_count[i] / total_people)) total_people -= stage_count[i] else: result.append((i, 0)) result.sort(key=lambda x: (-x[1], x[0])) return [x for x, _ in result] https:..